前回の記事の続きです。
では前回のコードに下記の様に音声をテキストに変換したあとに読み上げる機能を追加します。
import './style.css'; document.querySelector('#app').innerHTML = ` <div> <button id="startBtn">音声入力開始</button> <h5>結果</h5> <div id='result'></div> <button id="speakBtn">読み上げる</button> </div> `; // ブラウザがWeb Speech APIをサポートしているかを確認 if ('SpeechRecognition' in window || 'webkitSpeechRecognition' in window) { // SpeechRecognitionオブジェクトを作成 const recognition = new (window.SpeechRecognition || window.webkitSpeechRecognition)(); // 言語を日本語に設定 recognition.lang = 'ja-JP'; // 認識された結果を表示する要素を取得 const resultElement = document.getElementById('result'); const startBtn = document.getElementById('startBtn'); const speakBtn = document.getElementById('speakBtn'); // 認識されたテキストを取得したときの処理 recognition.onresult = (event) => { const transcript = event.results[0][0].transcript; resultElement.textContent = transcript; }; // 認識エラーが発生したときの処理 recognition.onerror = (event) => { console.error('音声認識エラー', event.error); }; // 音声認識を開始するイベントリスナーを追加 startBtn.addEventListener('click', () => { recognition.start(); }); // 音声認識を停止する関数 function stopRecognition() { recognition.stop(); } // 音声を読み上げる関数 function speakText() { const text = resultElement.textContent; if (text !== '') { const utterance = new SpeechSynthesisUtterance(text); utterance.lang = 'ja-JP'; speechSynthesis.speak(utterance); } } // 読み上げるボタンのクリックイベントリスナーを追加 speakBtn.addEventListener('click', speakText); } else { console.error('Web Speech APIはこのブラウザでサポートされていません'); }
追加された部分は次のとおりです:
import './style.css'; document.querySelector('#app').innerHTML = ` <div> <button id="startBtn">音声入力開始</button> <h5>結果</h5> <div id='result'></div> </div> `;
この部分では、style.css
ファイルをインポートし、#app
セレクターに対してHTMLコンテンツを設定しています。具体的には、ボタン、見出し、結果表示用の <div>
要素を含むHTMLを動的に生成しています。
// 音声認識を停止する関数 function stopRecognition() { recognition.stop(); }
この関数は、音声認識を停止するために recognition
オブジェクトの stop()
メソッドを呼び出します。
以上の追加された部分は、スタイルの読み込みと表示要素の生成、音声認識の言語設定、音声認識の停止処理に関連しています。これにより、適切なスタイルが適用されたHTMLが表示され、日本語の音声認識が行われ、必要に応じて音声認識が停止されます。
これで、音声入力されたテキストを読み上げてくれるようになりました。
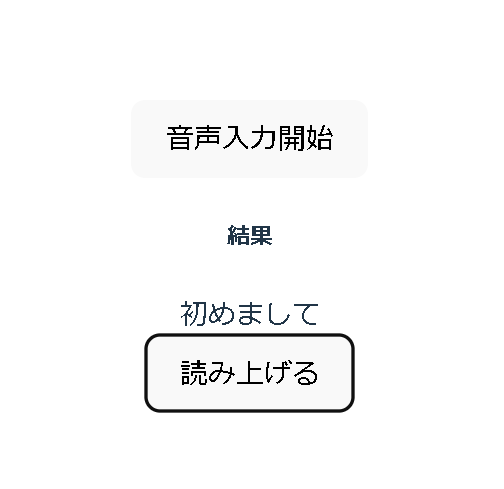
しかし!日本語で喋った内容をテキストにするのは理解できますがそれを再度スピーチさせる意図があまり考えられませんね。
ですのでGoogleの翻訳API(Google Cloud Translation API)などを使って英語でスピーチさせるなどを考慮していくと楽しいことになりそうです。